Interfacing the IoT: Automated Lighting with ESP8266 and Kotlin's Jetpack Compose & Ktor
/* by Vishal Gheravada - Jun 29, 2023 */
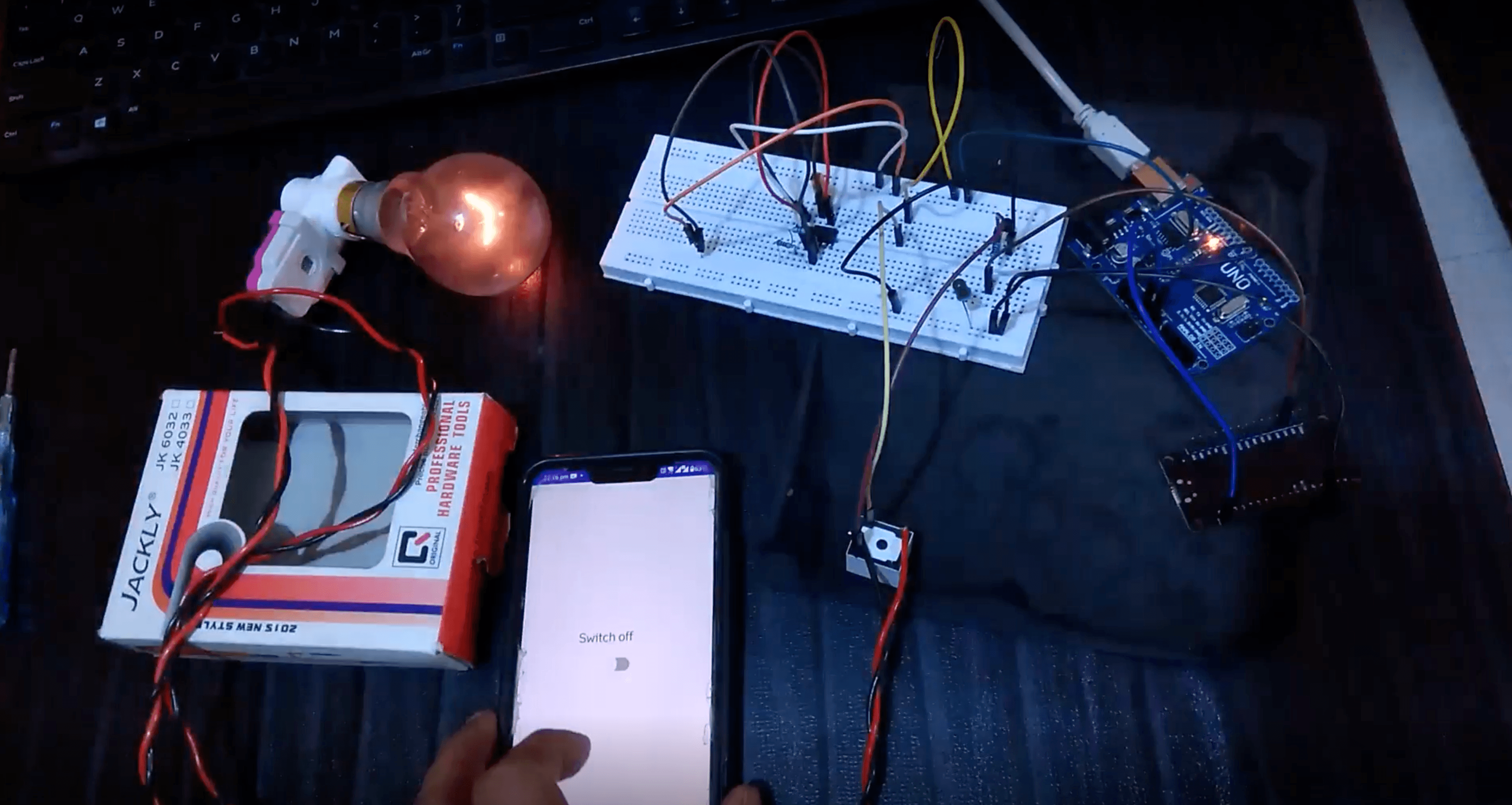
Introduction
In an era dominated by the Internet of Things (IoT), objects we interact with daily are becoming intelligent entities. With this in mind, today we're going to delve into the process of transforming an ordinary light bulb into a "smart bulb," manipulated through a custom Android application. Our tools of choice are the ESP8266 WiFi module, and an Android app using Kotlin, Jetpack Compose, and Ktor.
Part 1: Assembling the ESP8266 WiFi Module
ESP8266, a budget-friendly WiFi module, provides the means for microcontrollers to integrate with a WiFi network, enabling uncomplicated TCP/IP connections. This makes it an optimal choice for IoT-based undertakings. To automate our light bulb, we'll require:
- ESP8266 WiFi Module
- LED Bulb
- Relay Module
- Breadboard and Jumper Wires
- Power Supply
Establish a connection between the VCC and GND pins of the ESP8266 and the power supply, and then connect the GPIO2 pin to the relay module's input pin. Acting as an electronic switch, the relay module toggles the light bulb's state depending on the ESP8266's signal.
Part 2: Coding the ESP8266
The Arduino IDE simplifies the process of composing and uploading code to the ESP8266 module.
Arranging the Arduino IDE
- Fetch and install the latest version of the Arduino IDE.
- Include the ESP8266 board package in the Arduino IDE configurations.
- Find and add the ESP8266 board package from the Boards Manager.
Composing the Code
#include
#include
const char* ssid = "YourNetworkName";
const char* password = "YourNetworkPassword";
ESP8266WebServer server(80);
void setup(){
WiFi.mode(WIFI_AP);
WiFi.softAP(ssid, password);
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
int state = request->getParam(0)->value().toInt();
digitalWrite(2, state);
request->send(200, "text/plain", "Bulb state manipulated");
});
server.begin();
}
void loop(){
server.handleClient();
}
This script initiates a web server that awaits incoming connections and reacts based on the received requests.
Part 3: Android Application with Kotlin, Jetpack Compose, and Ktor
Jetpack Compose, a contemporary toolkit for crafting native Android UI, Kotlin, a statically typed programming language, and Ktor, a Kotlin asynchronous servers and clients building framework, are our selection for developing the Android application.
Preparing Android Studio
- Install the latest version of Android Studio.
- Start a fresh project with an empty activity template.
- Select Kotlin as the language and designate the minimum API level as per your requirement.
Designing User Interface with Jetpack Compose
Craft two buttons for controlling the light within the primary activity file.
@Composable
fun LightControlScreen() {
Column {
Button(onClick = { sendCommand("on") }) {
Text("Illuminate")
}
Button(onClick = { sendCommand("off") }) {
Text("Dim")
}
}
}
Configuring Ktor and Dispatching HTTP Requests
Incorporate Ktor dependencies in your build.gradle file:
dependencies {
implementation 'io.ktor:ktor-client-core:1.6.1'
implementation 'io.ktor:ktor-client-android:1.6.1'
}
Afterward, author the function that dispatches HTTP requests to the ESP8266 module:
val client = HttpClient(Android)
suspend fun sendCommand(state: String) {
val url = "http:///?state=$state"
val response: String = client.get(url)
}
Make sure to replace "<ESP8266 IP address>" with the actual IP address of your ESP8266 module.
Invoke the sendCommand function from a Coroutine scope as follows:
Copy code
Button(onClick = { CoroutineScope(Dispatchers.IO).launch { sendCommand("on") } }) {
Text("Illuminate")
}
Button(onClick = { CoroutineScope(Dispatchers.IO).launch { sendCommand("off") } }) {
Text("Dim")
}
Permissions
Don't forget to grant the internet permission in your AndroidManifest.xml:
Wrap-up
In this blog post, we journeyed through the procedure of creating an automated light control mechanism employing the ESP8266 WiFi module and an Android app developed with Kotlin, Jetpack Compose, and Ktor. IoT ventures such as this contribute to the growing trend of smart homes and also inspire novice developers to explore and create their intelligent devices. Happy coding!
References:
- ESP8266 WiFi Module Documentation
- Arduino IDE Documentation
- Android Studio Documentation
- Jetpack Compose Documentation
- Ktor Documentation
Conclusion
In this article, we detailed the process of creating an automated light control system using the ESP8266 WiFi module and an Android app developed with Kotlin, Jetpack Compose, and Ktor. IoT projects like this not only contribute to the growing smart home trend, but they also open the door for budding developers to tinker with and create their smart devices. Happy coding!