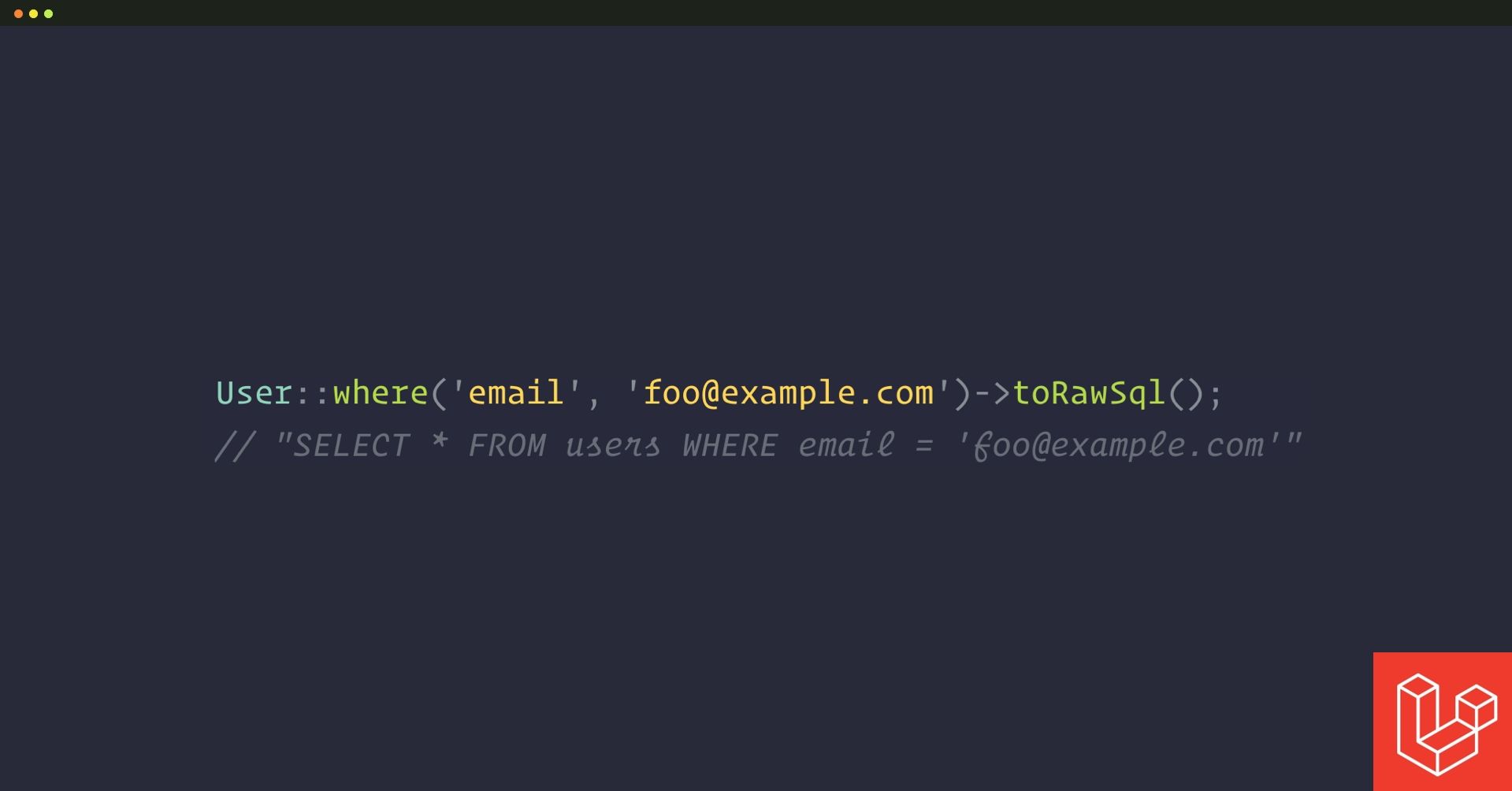
Laravel continues to impress with its introduction of developer-friendly features. One such game-changer in Laravel 10.x is the toRawSql()
method. This feature enables developers to print raw SQL queries alongside their bindings, significantly easing the debugging and optimization processes.
A Ride-Hailing Application Scenario
Imagine you're developing a ride-hailing application and need to fetch complex user ride data. Here's how you might construct the query using Laravel's Eloquent:
$rides = Ride::where('user_id', 1)
->where('created_at', '>=', now()->subMonth())
->where('cost', '>', 20)
->orderBy('distance', 'desc')
->toRawSql();
dd($rides);
With the toRawSql() method, this produces:
select * from `rides` where `user_id` = 1 and `created_at` >= '2023-06-12' and `cost` > 20 order by `distance` desc
Contrast this with the output from the same Eloquent query using dd()
in earlier Laravel versions:
$rides = Ride::where('user_id', 1)
->where('created_at', '>=', now()->subMonth())
->where('cost', '>', 20)
->orderBy('distance', 'desc')
->dd();
This produces:
"select * from `rides` where `user_id` = ? and `created_at` >= ? and `cost` > ? order by `distance` desc"
array:3 [
0 => 1
1 => "2023-06-12"
2 => 20
]
Taking Full Advantage of toRawSql()
As you can see, the toRawSql()
method is invaluable when handling complex queries. It allows you to easily view the exact SQL query that will be executed, with all bindings intact. This not only saves time but also eliminates the risk of errors that might occur during manual replacements.
Moreover, Laravel 10.x also introduces ddRawSql()
and dumpRawSql()
. These supplementary methods are a boon for developers, making it even more straightforward to print raw SQL queries directly.
Conclusion
The introduction of the toRawSql() method in Laravel 10.x is a testimony to the framework's continuous effort to provide developers with tools that make coding more efficient and enjoyable. As Laravel keeps evolving, it promises an exciting journey for both seasoned developers and beginners, one that is marked with continual learning and enhanced coding experience. Whether you are building a library system or a sophisticated ride-hailing application, Laravel 10.x is geared up to make your coding adventure seamless and pleasurable.